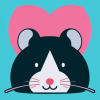
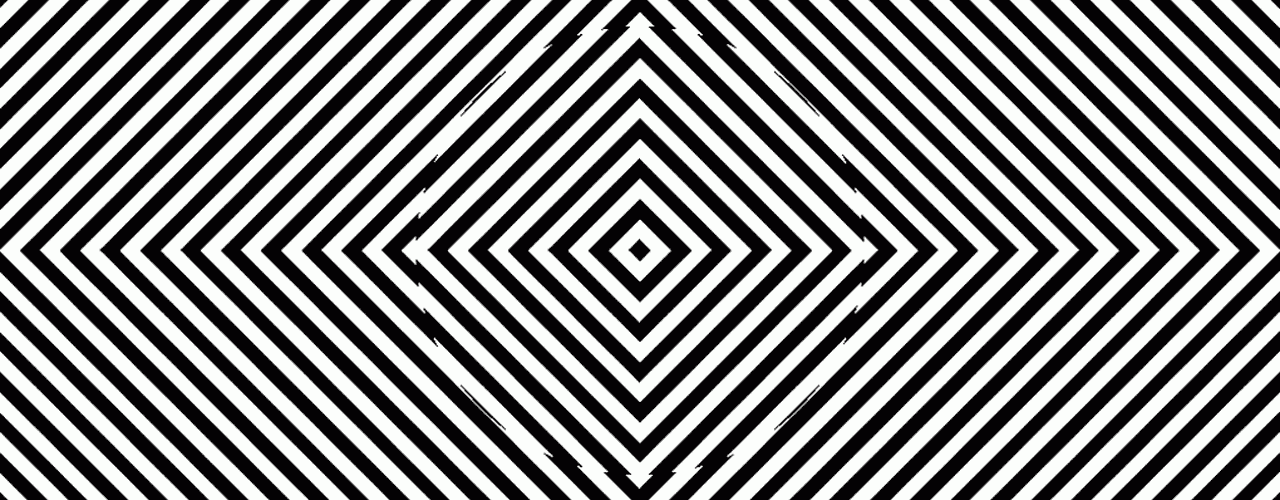
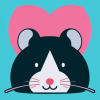
Estudante de engenharia eletrônica
This profile is from a federated server and may be incomplete. Browse more on the original instance.
Estudante de engenharia eletrônica
This profile is from a federated server and may be incomplete. Browse more on the original instance.